Solved
Medium
Topics
Companies
Hint
Given a 0-indexed n x n
integer matrix grid
, return the number of pairs (ri, cj)
such that row ri
and column cj
are equal.
A row and column pair is considered equal if they contain the same elements in the same order (i.e., an equal array).
Example 1:
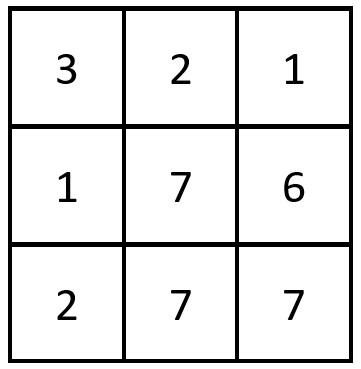
Input: grid = [[3,2,1],[1,7,6],[2,7,7]] Output: 1 Explanation: There is 1 equal row and column pair: - (Row 2, Column 1): [2,7,7]
Example 2:
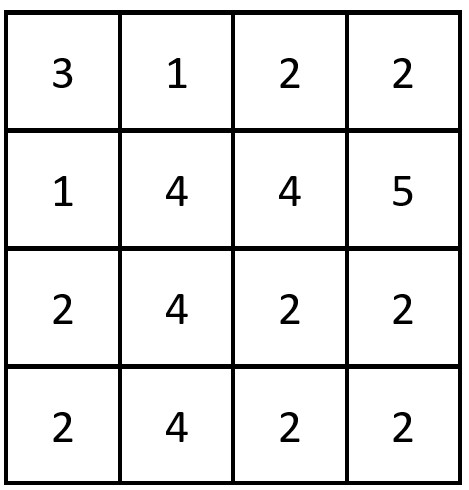
Input: grid = [[3,1,2,2],[1,4,4,5],[2,4,2,2],[2,4,2,2]] Output: 3 Explanation: There are 3 equal row and column pairs: - (Row 0, Column 0): [3,1,2,2] - (Row 2, Column 2): [2,4,2,2] - (Row 3, Column 2): [2,4,2,2]
Constraints:
n == grid.length == grid[i].length
1 <= n <= 200
1 <= grid[i][j] <= 105
google 翻譯:
給定一個 0 索引的 n x n 整數矩陣網格,傳回行 ri 和列 cj 相等的對 (ri, cj) 的數量。
如果行和列對包含相同順序的相同元素(即相等的陣列),則它們被視為相等。
想法一:
分兩個 hash 陣列
第一個 hash 存放"橫行"的數值
第二個 hash 存放"直列"的數值
最後再判斷內容相同個數
寫法一:
int equalPairs(int** grid, int gridSize, int* gridColSize) {
int **hash_row = malloc(sizeof(int *) * gridSize); // "橫行"的數值
int **hash_col = malloc(sizeof(int *) * gridSize); // "直列"的數值
int ret = 0;
for(int i=0; i<gridSize; i++) {
hash_row[i] = malloc(sizeof(int) * gridSize); // 存放內容的記憶體空間
hash_col[i] = malloc(sizeof(int) * gridSize); // 存放內容的記憶體空間
for(int j=0; j<gridSize; j++) {
hash_row[i][j] = grid[i][j]; // 存放"橫行"內容
hash_col[i][j] = grid[j][i]; // 存放"直列"內容
}
}
// 判斷 ”橫行“ 和 ”直列“ 兩陣列內容相同個數
for(int i=0; i<gridSize; i++) {
for(int j=0; j<gridSize; j++) {
if(!memcmp(hash_row[i], hash_col[j], sizeof(int) * gridSize))
ret++;
}
}
return ret;
}
結果一:

沒有留言:
張貼留言